Let say your web application needs to provide different user interface to different user groups. Or, you want to add additional modules without need to re-deploy complete application. In both example cases, one of the ways to achieve this is by loading of user controls dynamically.
Create a new project with one web page and add PlaceHolder control to web form. Markup code of web page could look like this:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="DefaultCS.aspx.cs" Inherits="DefaultCS" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:PlaceHolder ID="phModule" runat="server"></asp:PlaceHolder>
</div>
</form>
</body>
</html>
Now add one web user control to project and name it "MyUserControl.ascx". Add some content to user control. This code example contains one Label control with welcome message:
<%@ Control Language="VB" AutoEventWireup="false" CodeFile="MyUserControl.ascx.vb" Inherits="MyUserControl" %>
<asp:Label ID="Label1" runat="server"
Text="Hello, this is My User Control dynamically loaded :)"></asp:Label>
Now, you can write ASP.NET server side code to web page, to dynamically load web user control. Code could look like this:
[ C# ]
// We need this namespace to load controls dynamically
using System.Web.UI;
public partial class DefaultCS : System.Web.UI.Page
{
protected void Page_Load(object sender, System.EventArgs e)
{
// Load control from file "MyUserControl.ascx"
Control myUserControl =(Control)Page.LoadControl("MyUserControl.ascx");
// Place web user control to place holder control
phModule.Controls.Add(myUserControl);
}
}
[ VB.NET ]
' We need this namespace to load controls dynamically
Imports System.Web.UI
Partial Class _Default
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
' Load control from file "MyUserControl.ascx"
Dim myUserControl As Control = Page.LoadControl("MyUserControl.ascx")
' Place web user control to place holder control
phModule.Controls.Add(myUserControl)
End Sub
End Class
Finally, you can start an example and enjoy in dynamically loaded web user control, like in image bellow:
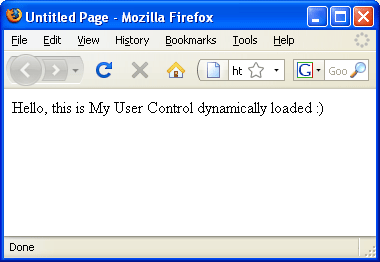
Web user control loaded at run time
Happy programming!
2 comments:
Very useful instructions! This is EXACTLY what I was looking for. Simple and elegant solution to dynamically creating a group of controls.
Do you have a post explaining how to gather the data entered into a user control that was placed in a placeholder?
Example: I have 4 textboxes in the user control and I want to put the values entered from 4 of the user controls dynamically created into a database. (So 16 values to store in a database)
Thanks again for a great example
Thanks and i will look into this.....
Post a Comment